本文共 581 字,大约阅读时间需要 1 分钟。
2021.5.9
目前在做答辩PPT,还有两天就要答辩了,我有点担心自己写的太简单过不了…除了我,谁还想到用C++写?
把简单的事情做到极致,把想表达的意思表达清楚,自己的论文多看、多修改、理解自己写的框架,论文再怎么次也是自己写的孩子,总不能对自己不自信吧。加油吧,我还有开题报告要写,好羡慕室友她们不用做PPT。。。哎。。。过几天还得再参加教资面试,,真的太累了。。。。我发现我就是喜欢给自己找事做。。。闲下来就觉得自己是个废物。。。。
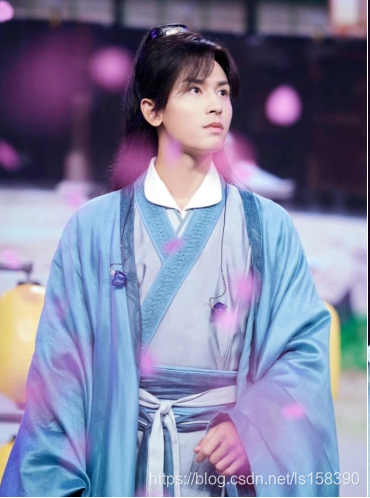
最近太磕ZZH,愿星途闪耀,归来仍是少年。青山不改,绿水长流,天涯路远,终有重逢之际,愿平行空间里的周子舒和温客行亦可诗酒江湖,知己常在。
2021.5.10 答辩PPT已经做好了,表格也做好了。。明天就要答辩了。。今天去找导师看见同组的同学做的系统,对比之下觉得自己做的好差。。差就差吧,总要面对。。下午还要做兼职,现在的心情七上八下。。
1.打印论文3份
2.答辩PPT
3.开题报告
4.演示系统 答辩稿
别浪了。。。好好看自己的论文吧,格式,排版什么的,希望别出大问题。。。
下午16:28
我去,下了好大的雨加冰雹,兼职不去了。忽然想起来我是未来三年要去武汉上学的。。。悲伤辣么大。。信阳这的天气太吓人了,一直电闪雷鸣的,楼下得谁的电车被淋的呜呜响…
2021.5.11 倒计时两小时…加油。。。答辩完再更。。
转载地址:http://pngiz.baihongyu.com/